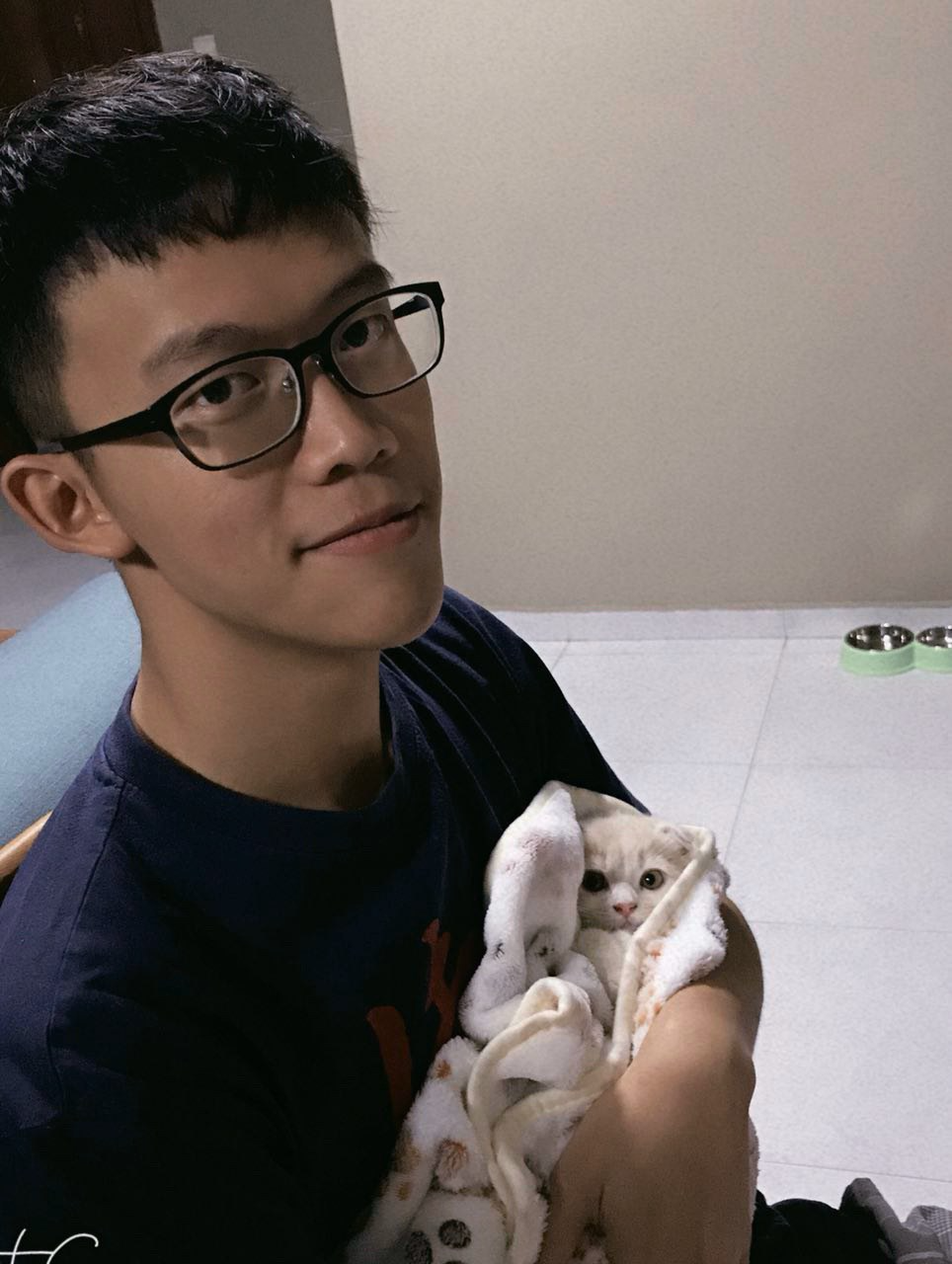
About this project
My 5-man strong team of software-engineering students were tasked to take a basic command line interface desktop application called, addressbook, and improve upon it. We came up with Task Manager which offers gamification elements. Apart from standard CRUD capabilities expected of a task manager, BBProductive includes a “Pomodoro” timer to help users do manageable work cycles. BBProductive also has a virtual pet that grows and changes depending on how much work the user has done.
Summary of contributions
-
Enhancements: I implemented the Pomodoro core feature of the app.
-
What it does: The “pom” command allows the user to activate the pomodoro feature. It takes a second input from the user, the index of a chosen task and starts a timer. This timer is set to 25 minutes by default but can be adjusted by the user via settings or for a single instance via the “tm/” prefix. Upon expiration of the timer, the app prompts users to check on their progress and updates the task manager accordingly. The app will also prompt if the user wants to begin the pomodoro-standard break time.
-
Justification: The Pomodoro technique is a productivity framework we decided to adopt as it has been championed by various sites and creatives. It was also voted one of the most popular techniques on LifeHacker.
-
Highlights: First, the basic command parser provided by AB3 gave me a good foundation to branch from. I had to adapt the parser to accept multiple types of input (i.e. I accept “pom 1” and “pom pause” for example). Second, building the actual pomodoro feature required me to use JavaFX’s Timeline class. The Timeline class adequately served my requirements for the Pomodoro work and rest cycles. Lastly, to handle prompts during pomodoro cycles, I decided to swap the “Command Executor” that exists in the Main Window class so I could better encapsulate Pomodoro related responses.
-
Relevant PRs: [#92], [#126], [#146, [#147], [#262], [#263], [#269]
-
-
Minor enhancement: I added a switch tabs command that allows the user to navigate to between the tabs.
-
Relevant PR: [#127]
-
-
My code contributions on RepoSense: [RepoSense]
-
Other contributions:
-
Documentation:
-
Given below are sections I contributed to the User Guide. |
Pomodoro (Hardy)
In this section, let’s learn how you can take advantage of the Pomodoro feature to boost your productivity! Learn all the commands you can use for Pomodoro.
What’s Pomodoro?
In the late 1980s, a gentleman named Francesco Cirillo devised a time management method called the Pomodoro Technique. Essentially, a single cycle consists of two parts, 25 minutes of work, followed by a 5 minutes break. This cycle repeats for as long as you want to get work done.
Let’s get started!
BBProductive’s Pomodoro feature is very easy to use. Let’s take a look at the following steps!
Step 1: pom
a task to get started!
You can activate the Pomodoro timer and set a task you want to focus on. The default timer for a work cycle is 25 minutes. However, you can add an optional timer amount field for a particular cycle.
Format: pom <index> [tm/<amount in minutes>]
Indexes refer to the current tasklist on display, it does not refer to the indices of the entire task list. Note that indexes start from 1 and not 0. You can input a value (decimals allowed) following the prefix |
After you’ve successfully pommed a task, you can see the task’s name in the bar at the top of the task list. The timer there will start counting down as well.
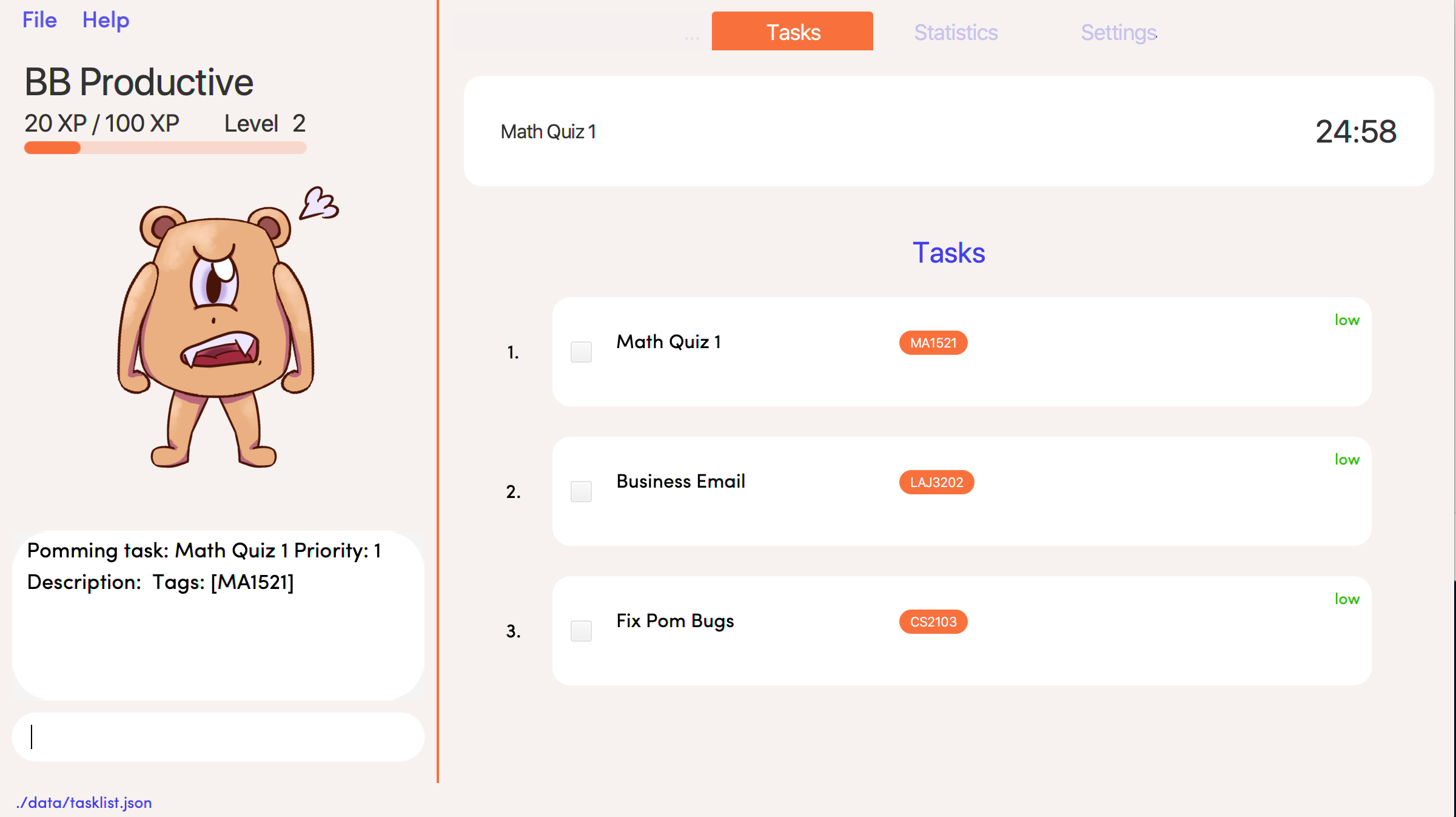
Step 2: pom pause
if you need to take a quick break.
Not exactly a break. But let’s say you need to leave your desk real quick. You can pause a running Pomodoro timer by simply calling pom pause
.
Once you’ve paused Pomodoro, the timer will freeze and remain so until you resume in the next step.
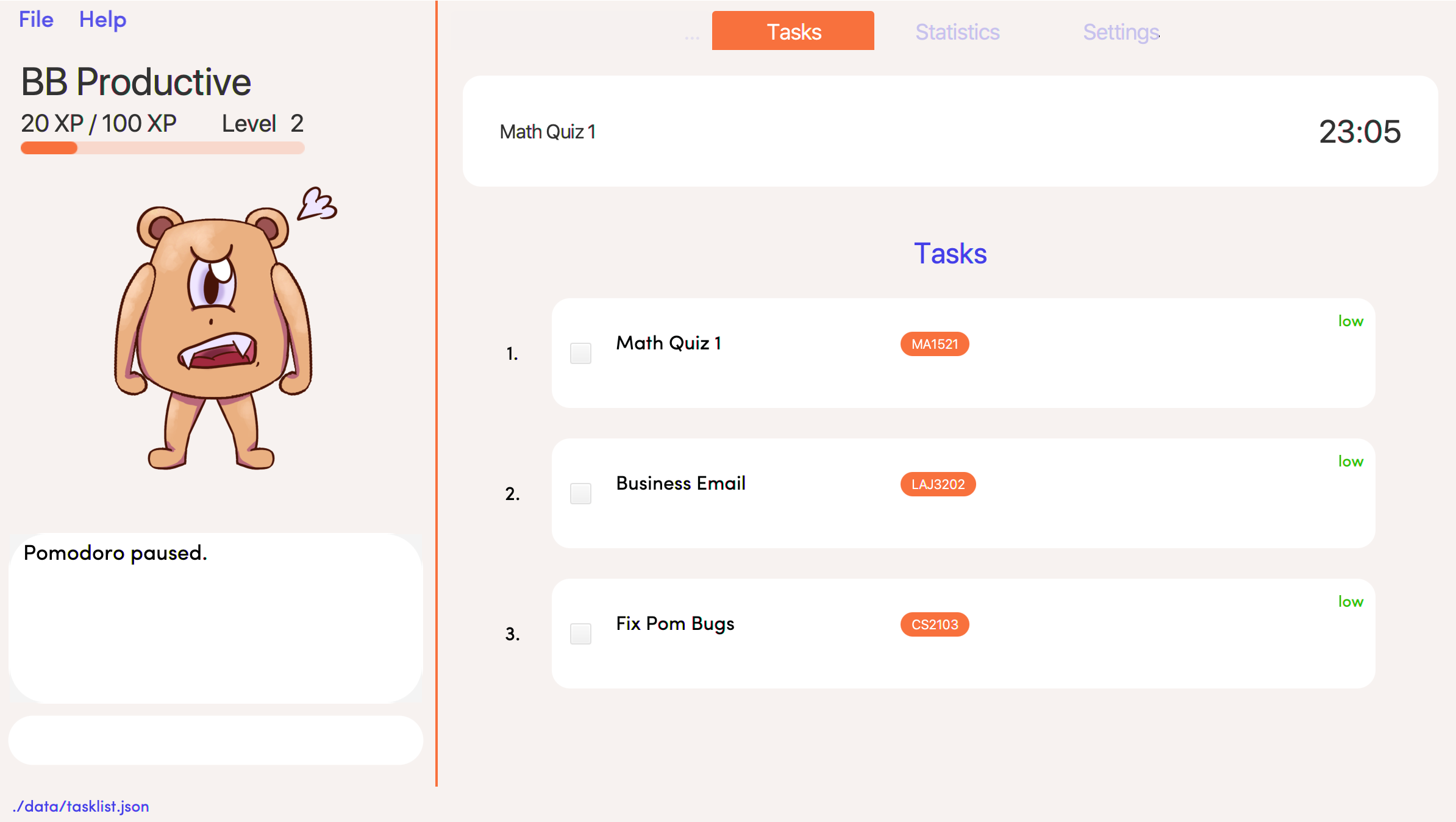
Step 3: pom continue
to get back to work.
Now that you’re back and ready to get back to work, simply use pom continue
to resume for where you left off.
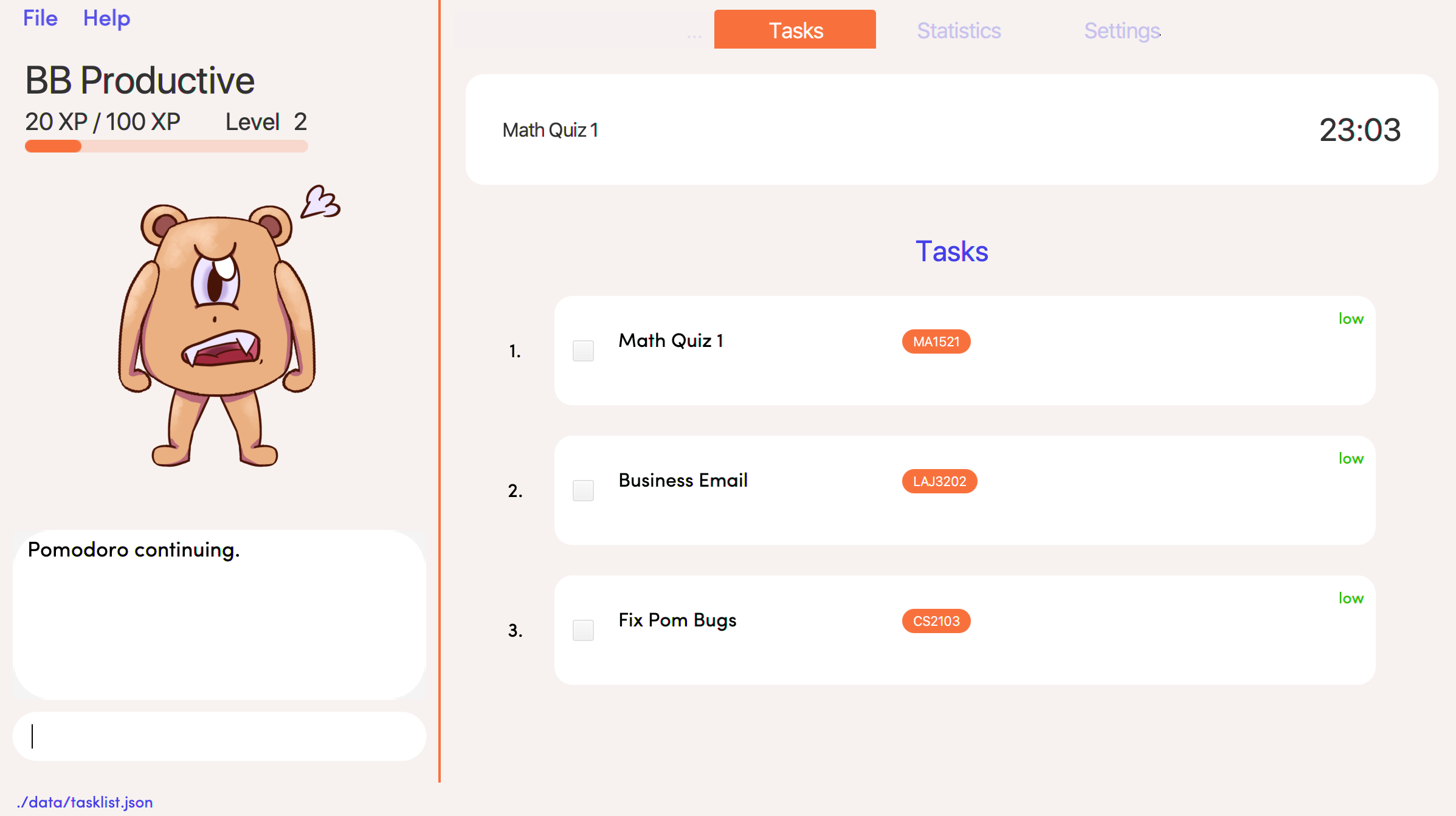
Step 4: Time’s up!
Once the timer expires, the app will prompt you if you have finished the task. You need only respond with Yes (Y) or No (N) in order to proceed.
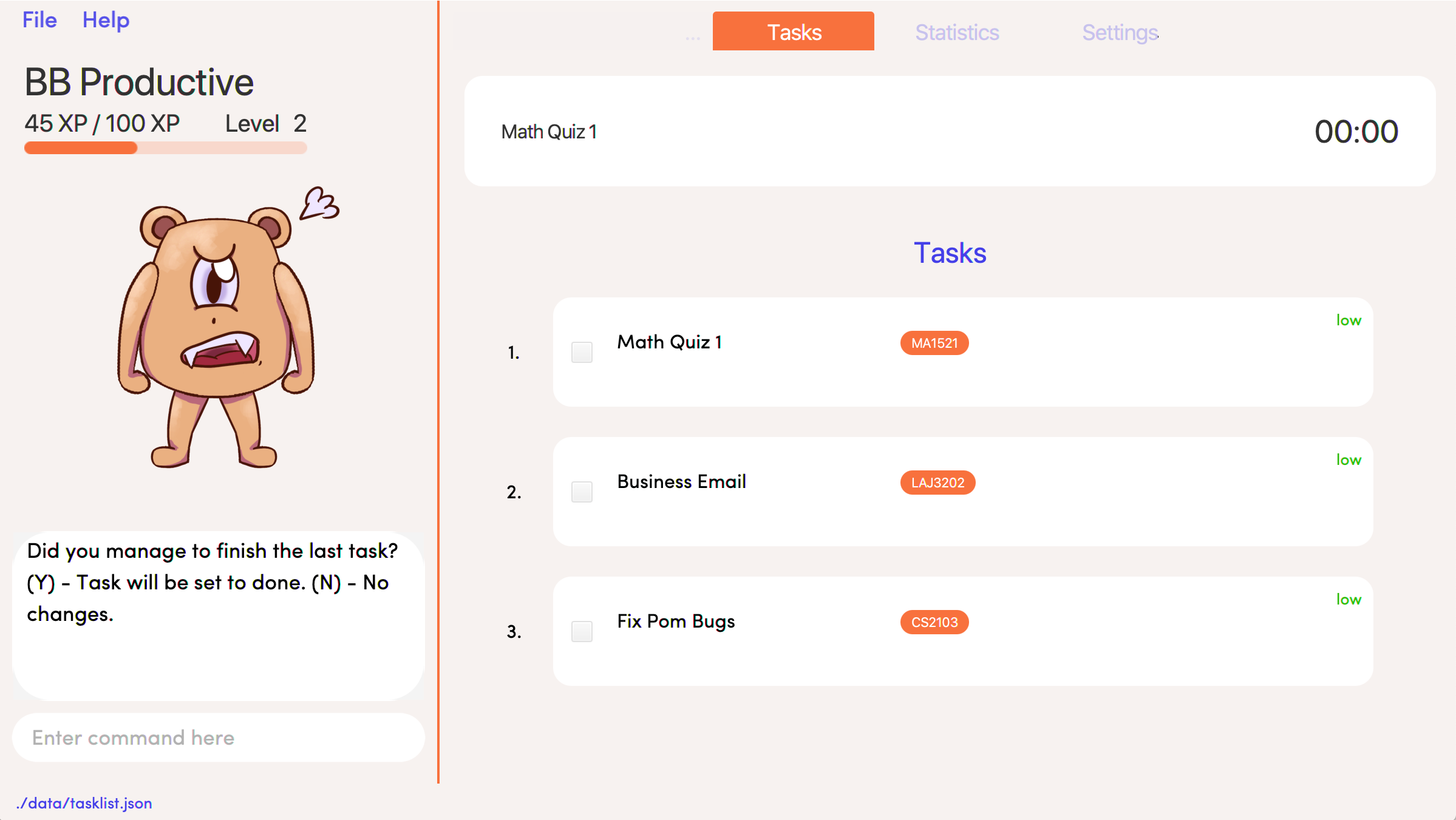
Step 5: Break-time!
Afterwards, the app will prompt you if you would like to begin a 5-minute break (as per the Pomodoro technique). Similarly, you need to respond with Yes (Y) or No (N). Responding with No(N) will set the app to its neutral state. Responding with a Yes(Y) will start the break timer.
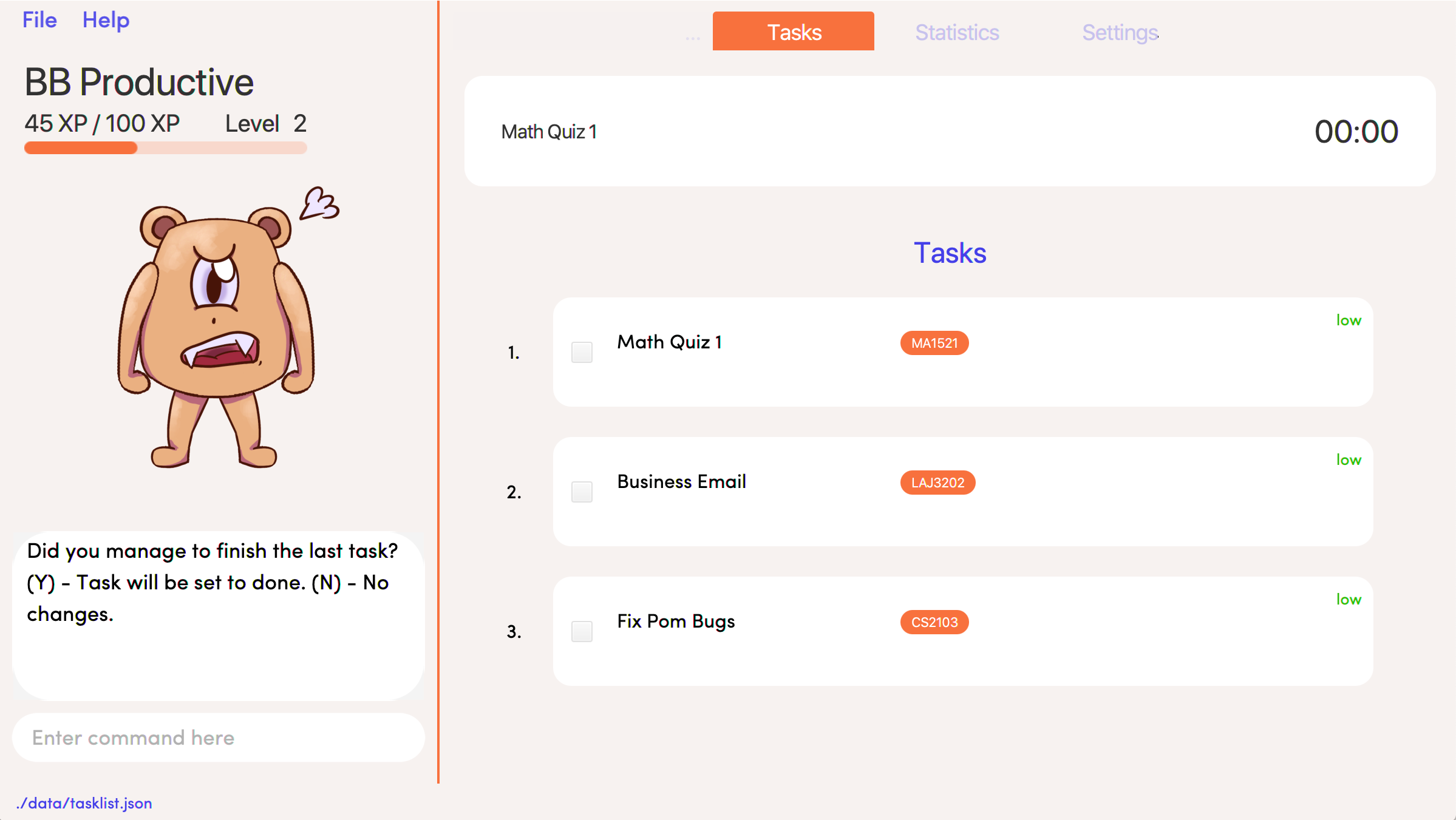
You will not be able to execute other commands during these prompts. Please respond to the prompts to proceed. |
Back to Step 1
At the end of the break, the app will return to its "neutral" state. Wish to start on another Pomodoro cycle? Head back to step 1 for another journey of productivity.
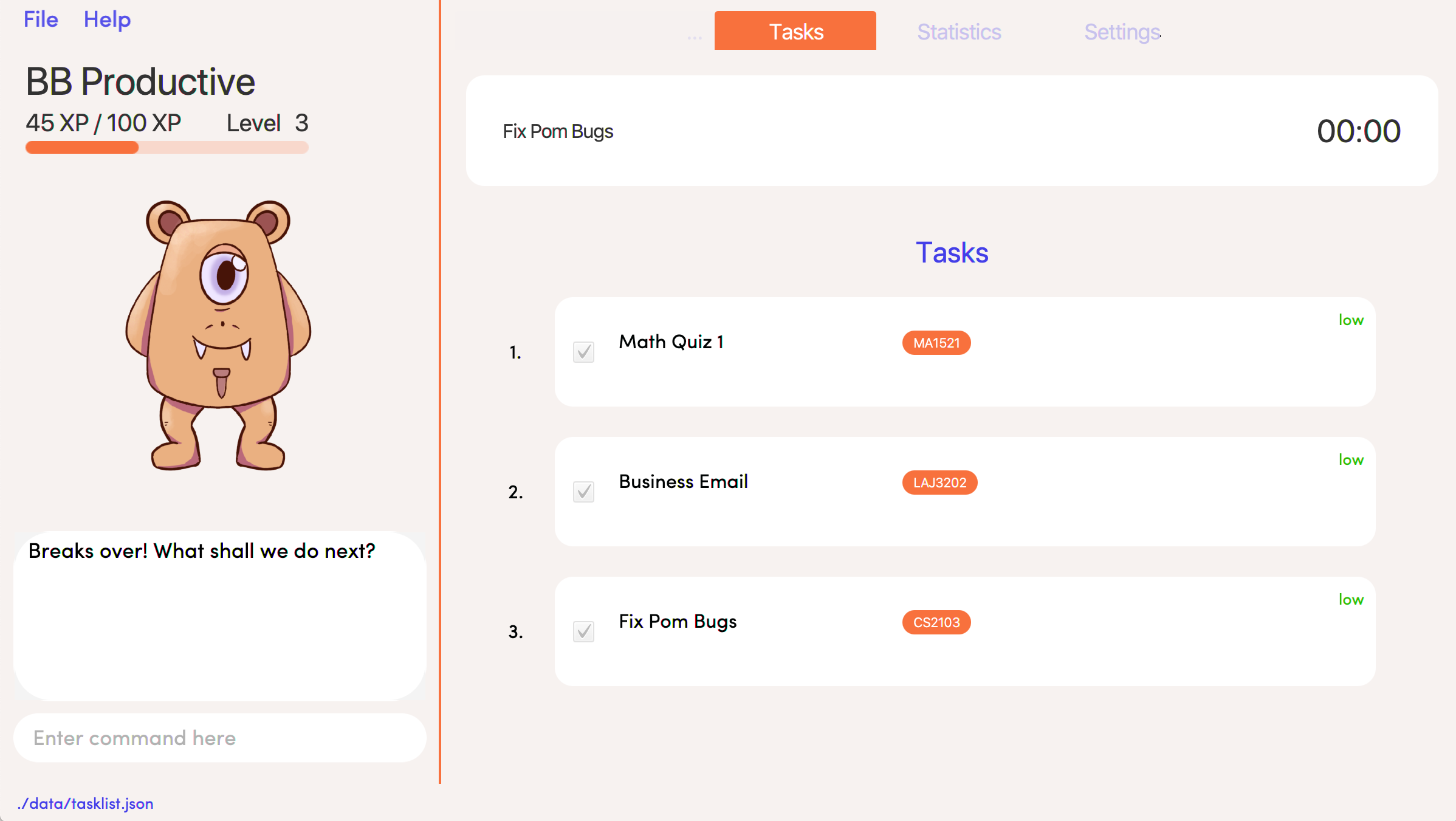
Command Table Summary (Hardy)
The following table summarizes all the commands that you can use. Input contained with in […] are optional fields.
Command | Format | Example |
---|---|---|
Add |
|
|
Edit |
|
|
Done |
|
|
Delete |
|
|
Pom |
|
|
find |
|
|
Tag |
|
|
Sort |
|
|
Tasks |
|
|
Stats |
|
|
Settings |
|
|
Set |
|
|
Clear |
|
|
I also contributed content to BBProductive’s Developer Guide.
Given below are sections I contributed to the Developer Guide. |
Pomodoro
Pomodoro is activated by the pom
command. It follows the same execution flow as many of the other commands in BBProductive.
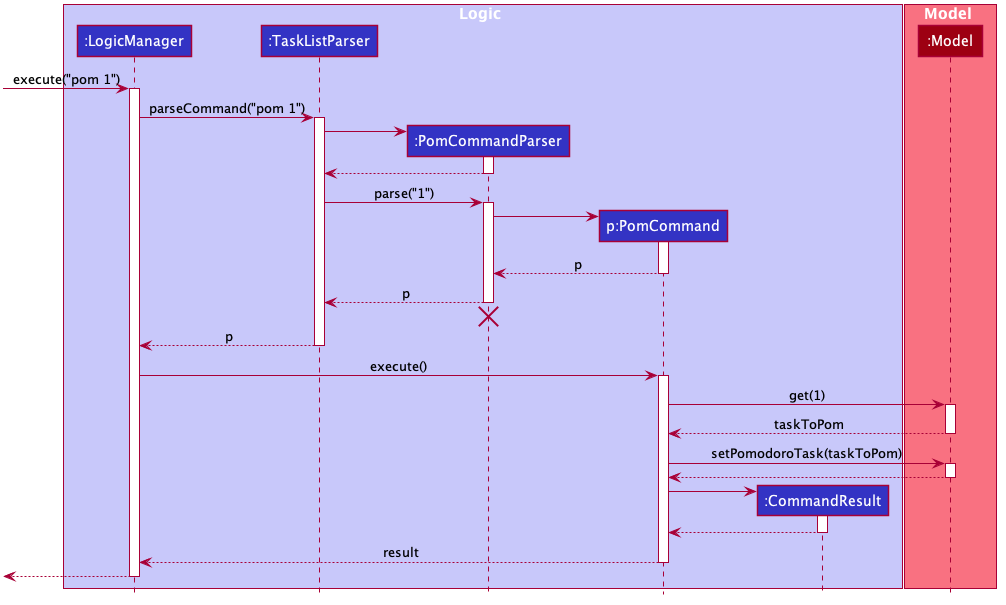
Implementation
Pomosoero’s features are implemented mainly in seedu.address.logic
package. The PomodoroManager
class is used to maniulate the timer and configure the relevant UI elements. The timer is facilitated by javafx.animation.Timeline
.
When the PomCommand
is executed, the PomodoroManager
will handle the actual timer systems and update the relevant entities in the app. This is evident in the following sequence diagram.
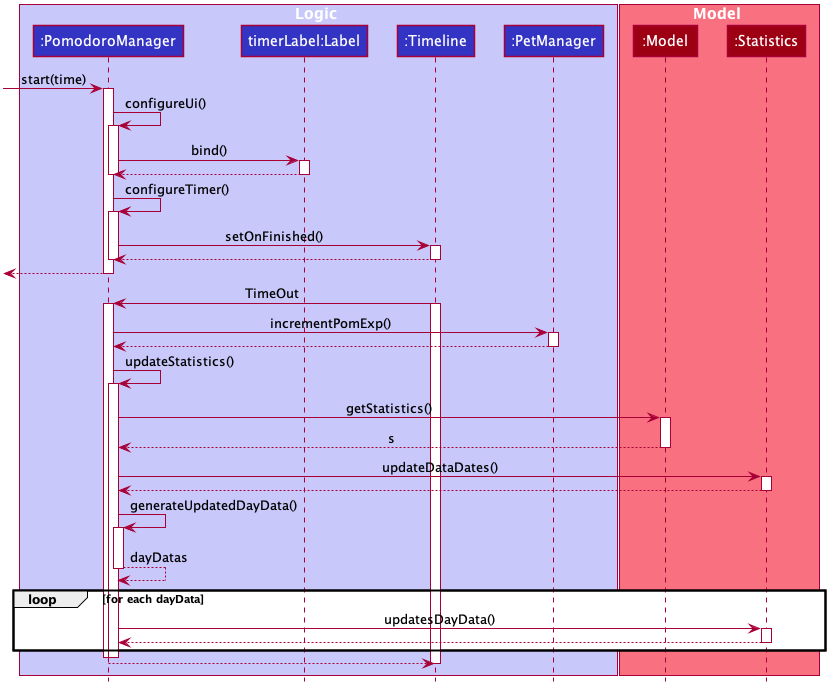
Through the use of the Pomodoro feature, there are occasions where the app has to prompt the user for specific input in order to progress. This behaviour flow is represented in the Pomodoro Acctivity diagram.
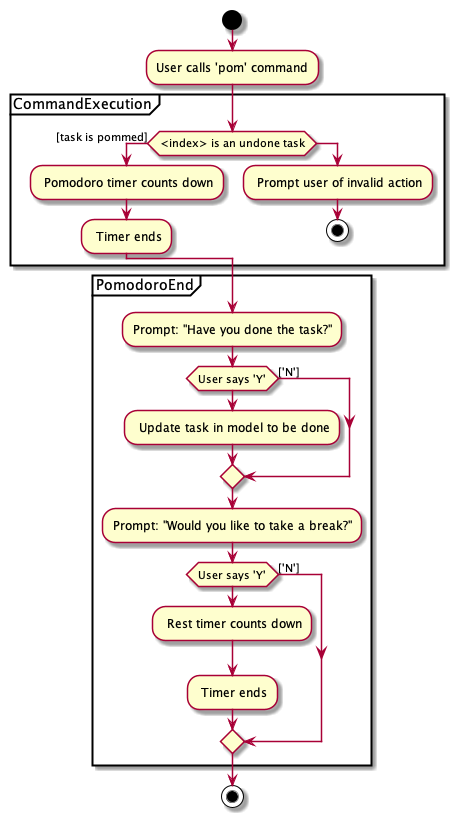
The PomodoroManager
maintains a prompt_state
indicating what the app might be prompting the user at a given time.
Pomodoro Prompt States
-
NONE
: There is no particular prompt happening. The default state when the app is in the neutral state. (i.e. No pomodoro running.) -
CHECK_DONE
: This state occurs when a timer expires during a Pomodoro cycle. -
CHECK_TAKE_BREAK
: This state occurs after user response has been received in the CHECK_DONE state. -
CHECK_DONE_MIDPOM
: This state occurs when the user calls done on a task that is the Pomodoro running task.
Pomodoro has settings that can be configured by the user:
-
Pomodoro Time: This defines how long the Pomodoro work period is. The default is 25 minutes.
-
Break Time: This defines how long the breaks last in between Pomodoro periods. The default is 5 minutes.
This data is captured and stored in the Pomodoro
class in seedu.address.model
, which interacts with the app’s storage system. PomodoroManager
also updates the Pomodoro
model on what task is being run and the time remaining in a particular cycle. This allows the time progress to be persistent in between app closures and relaunches.
Use Cases
(For all use cases below, the System is BBProductive
and the Actor is the user
, unless specified otherwise)
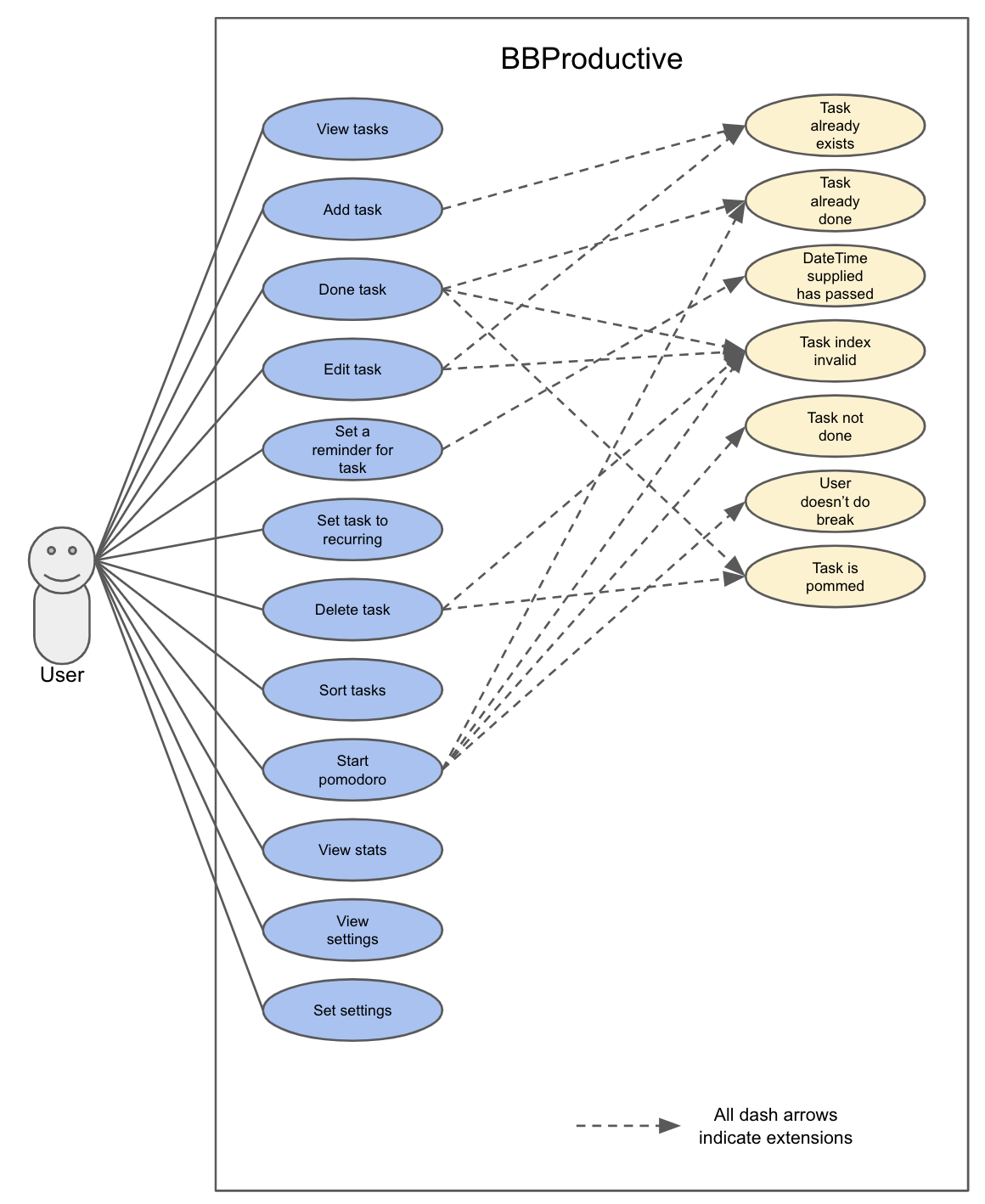
Use Case: UC01 - View tasks
MSS
-
User requests to see the task list.
-
BB Productive displays the view under the tasks tab.
Use case ends.
Use Case: UC02 - Add task
MSS
-
User requests to add a task to the task list.
-
BB Productive shows view with updated task list.
Use case ends.
Extensions
-
1a. Task of the same name already exists.
-
1a1. BBProductive shows "This task already exists in the task list" in response box.
Use case ends.
-
Use Case: UC03 - Done task
MSS
-
User requests to set a task to done.
-
BB Productive shows view with updated task list.
Use case ends.
Extensions
-
1a. Task specified by user already marked as done.
-
1a1. BBProductive shows "Task has already been marked as done!" in response box.
Use case ends.
-
-
1b. User fed in an invalid index.
-
1b1. BBProductive shows "Invalid command format! " in response box.
Use case ends.
-
-
2a. A pommed task is among the tasks to be set to done.
-
2a1. BBProductive prompts user if they want to
pom
another task, orN
to return the app to neutral. -
2a2. If user
pom
another task, use case resumes at stage 2 of UC09.Use case ends.
-
Use Case: UC04 - Edit task
MSS
-
User requests to update a task with updated fields and informs the task list.
-
BB Productive shows view with updated task list.
Use case ends.
Extensions
-
1a. New task name matches that of another task.
-
1a1. BBProductive shows "This task already exists in the task list." in response box.
Use case ends.
-
-
1b. User fed in an invalid index
-
1b1. BBProductive shows "Invalid command format! " in response box.
Use case ends.
-
Use Case: UC05 - Set a reminder for a task
MSS
-
User requests to set a task with a Reminder.
-
BB Productive creates/updates a task and shows the view with updated task list.
-
A reminder pops up when the specified time has elapsed.
Use case ends.
Extensions
-
1a. New task name matches that of another task.
-
1a1. BBProductive shows "This task already exists in the task list." in response box.
Use case ends.
-
Use Case: UC06 - Set a task to recurring
MSS
-
User requests to set a task to be a recurring task.
-
BB Productive creates/updates a task and shows the view with updated task list.
-
A reminder pops up when the specified time has elapsed.
Use case ends.
Extensions
-
1a. New task name matches that of another task.
-
1a1. BBProductive shows "This task already exists in the task list." in response box.
Use case ends.
-
Use Case: UC07 - Delete task
MSS
-
User requests to list tasks.
-
BBProductive shows a list of tasks.
-
User requests to delete a specific person in the list.
-
BBProductive deletes the task.
Use case ends.
Extensions
-
1a. New task name matches that of another task.
-
1a1. BBProductive shows "This task already exists in the task list." in response box.
Use case ends.
-
-
1b. User fed in an invalid index.
-
1b1. BBProductive shows "Invalid command format!" in response box.
Use case ends.
-
-
1c. Task to be deleted is being pommed.
-
1c1. BBProductive shows "You can’t delete a task you’re pom-ming!" in response box.
Use case ends.
-
Use Case: UC08 - Sort tasks
MSS
-
User requests to list tasks.
-
BBProductive shows a list of tasks.
-
User requests to sort the list by one or more parameters.
-
BBProductive creates a new view and updates the task list view.
Use case ends.
Use Case: UC09 - Start pomodoro
MSS
-
User requests to start pomodoro on a specific task.
-
BBProductive starts timer and sets task-in-progress to said task.
-
Pomodoro timer expires.
-
BBProductive sets task-in-progress to null and prompts user if user has done the task.
-
User replies the affirmative.
-
BBProductive shows view with updated task list with done task. Pet adds additional points.
-
BBProductive prompts user if user wants to do break time.
-
User replies the affirmative.
-
BBProductive starts break timer.
-
Break timer expires.
-
BBProductive returns to neutral state.
Use case ends.
Extensions
-
1a. User fed in an invalid index.
-
1a1. BBProductive shows "Invalid command format! " in response box.
Use case ends.
-
-
1b. Task specified by user already marked as done.
-
1b1. BBProductive shows "Task has already been marked as done!" in response box.
Use case ends.
-
-
5a. User replies negative.
-
5a1. BBProductive will leave the task list as is.
Use case resumes at stage 7.
-
-
5b. User replies with answer that is neither
Y/y
norN/n
.-
5b1. BBProductive will leave the task list as is.
Use case resumes at stage 7.
-
-
8a. User replies negative.
-
8a1. BBProductive will start no timer.
Use case resumes at stage 11.
-
Use Case: UC10 - View stats
MSS
-
User requests to see the statistics tab.
-
BBProductive displays the view under the statistics tab.
Use case ends.
Use Case: UC11 - View settings
MSS
-
User requests to see the settings tab.
-
BBProductive displays the view under the settings tab.
Use case ends.
Use Case: UC12 - Set settings
MSS
-
User requests to update the app’s settings.
-
BBProductive takes the input and updates the app’s internal settings.
-
User requests to see the settings tab.
-
BBProductive displays the view under the settings tab with the updated preferences.
Use case ends.